Introduction to Node.js and Its Installation
- Anushka Shrivastava
- Aug 25, 2022
- 4 min read
Updated: Oct 6, 2022
Node.js is an open-source and cross-platform JavaScript runtime environment, which means, it is an open source server environment which runs on various platforms such as Windows, Linux, Mac OS, etc.
An open source web server, in the above definition, means it is a public-domain software designed to deliver web pages over World Wide Web (www).
In this article, we will be discussing about the basics of Node.js that a beginner should know, followed by it's installation process and some hands on coding. By the end of this article, you will be able to write your very first code of Hello World in Node.js Application.
Quick links -
Features of Node.js
Following are some of the most important features of Node.js :

1. Runs V8 JavaScript Engine – Node.js is a server-side platform that runs V8 JavaScript engine of Google Chrome, which increases its performance.
2. Does not create new thread for every request – A Node.js app runs in a single process without creating new thread for every request. Libraries in Node.js are written using non-blocking paradigms, which controls its blocking behavior otherwise.
3. Can handle multiple concurrent connections with single server – When Node.js performs an I/O operation, like accessing data from database or reading data from a file system, it does not block the thread and waste CPU cycles. Instead, it resumes the operations when response comes back. The process allows Node.js to handle multiple connections with single server without any burden.
4. Uses JavaScript as a programming language – There are many front end developers who write code in JavaScript for browser. They can write code for server-side as well in the same programming language, without having to learn a completely different language.
5. Supports vast number of libraries – Node.js uses npm package manager. Npm registry hosts over 1,000,000 open source packages which are free to use.
Where to use Node.js
Node.js has a vast range of applications. Some of the major areas where Node.js can be used are -
1. I/O bound applications – Any application that involves reading or/and writing data from an input-output system, as well as waiting for information, is considered I/O bound application. These include applications like web applications, downloading files, etc.
2. Data Streaming applications – Data streaming is the process of transmitting a continuous flow of data to derive valuable insights. Streamed data is generated continuously by thousands of data sources. Some of the examples where data streaming is used are :- real-estate websites, online gaming, media publishing, etc.
3. JSON API based applications – JSON (JavaScript Object Notation) API is an application programming interface designed for lightweight data interchange between two computer applications.
4. Single-page applications – A single page application (SPA) is a web application or website that interacts with user by dynamically rewriting the current web page with new data from web server, without loading the entire new web page. For example – real time chatting applications.
Now, let us move further and install Node.js
INSTALLATION OF NODE.JS
Node.js can be installed in different ways. The most common and convenient way to download and install it is from the official Node.js website which includes all the instructions - https://nodejs.org/en/
Another way is to install Node.js via nvm. The detailed instructions on how to install via this method is provided in the official documentation of node.js. You can refer to - https://nodejs.dev/en/learn/how-to-install-nodejs/
HELLO WORLD PROGRAM – An example of Node.js Application
After the installation process is completed, let us code our first program in Node.js, named helloWorld.js.
First, let us look at the code, and the output produced. The text editor used here is vscode.
const http = require('http')
const hostname = 'localhost'
const port = 3000
const server = http.createServer((req, res) => {
res.end('Hello World\n')
})
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`)
})
Type “node helloWorld.js” on terminal to connect to the server.
node helloWorld.js
The output we get from above code in terminal is -

And on clicking on the link http://localhost:3000/ , we get following as our output:
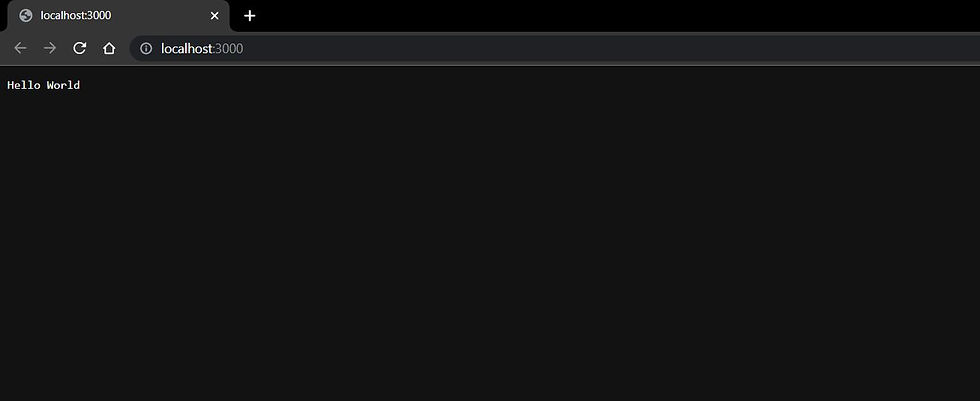
In the above code,
The code first includes the Node.js http module. It is a built-in module of Node.js, which is required to transfer data over the HTTP (Hyper Text Transfer Protocol) for networking.
const http = require('http')
Hostname and port number are then defined for the server.
const hostname = 'localhost'
const port = 3000
The createServer() method of http module, creates a new server and returns it to the constant variable server.
const server = http.createServer((req, res) => {
res.end('Hello World\n')
})
The server is set to listen on the specified hostname and port. When the server is ready, the callback function is called. In this case, it is informing us that the server is running on specified hostname and port.
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`)
})
Here, ()=>{.....} inside server.listen() method is defined as a callback function. It is a concept of JavaScript.
The “req, res” passed as arguments while creating server are two important objects to handle the HTTP call. The first provides the request details. It is not used this code example. The second one is used to return data to the caller. We close the response , adding the content as an argument to end().
res.end('Hello World\n')
With this, we come to the end of this article. Topics we covered are -
Thankyou.
Recent Posts
See AllNodemon is a very powerful and useful tool. Every time we make changes in our node.js program, we need to save it and then run it again...
Comments