JavaScript We Need To Know Before Node.js – PART 1
- Anushka Shrivastava
- Aug 28, 2022
- 6 min read
Updated: Oct 6, 2022
As Node.js uses JavaScript as its programming language, it is important to know about the basics to intermediate level of JavaScript. The official documentation of Node.js recommends to have a good grasp on the following concepts:
Lexical Structure
Expressions
Types
Classes
Variables
Functions
This
Arrow functions
Loops
Scopes
Arrays
Template literals
Semicolons
Strict mode
ECMAScript 6, 2016, 2017
In this article, we will be dealing with the following areas of concepts –
Other topics are covered in articles containing part-2 and part-3. You can refer to the following links for the same:
Let us try to understand and take an overview of above mentioned concepts for complete beginners to the subject.
LEXICAL STRUCTURE
JavaScript source text is just a sequence of characters for machines if not interpreted. In order for interpreter to understand the code and process it further, the sequence of characters or source text string has to be parsed to a more structured representation, so that further processes can take place.
In simple words, we can say that lexical structure of JavaScript or any other programming language specifies a set of some basic rules about how a code should be written, how variables should be defined, how a program statement should be separated from the next and list goes on.
Elements that are the basic building blocks for defining lexical structure of JavaScript are –
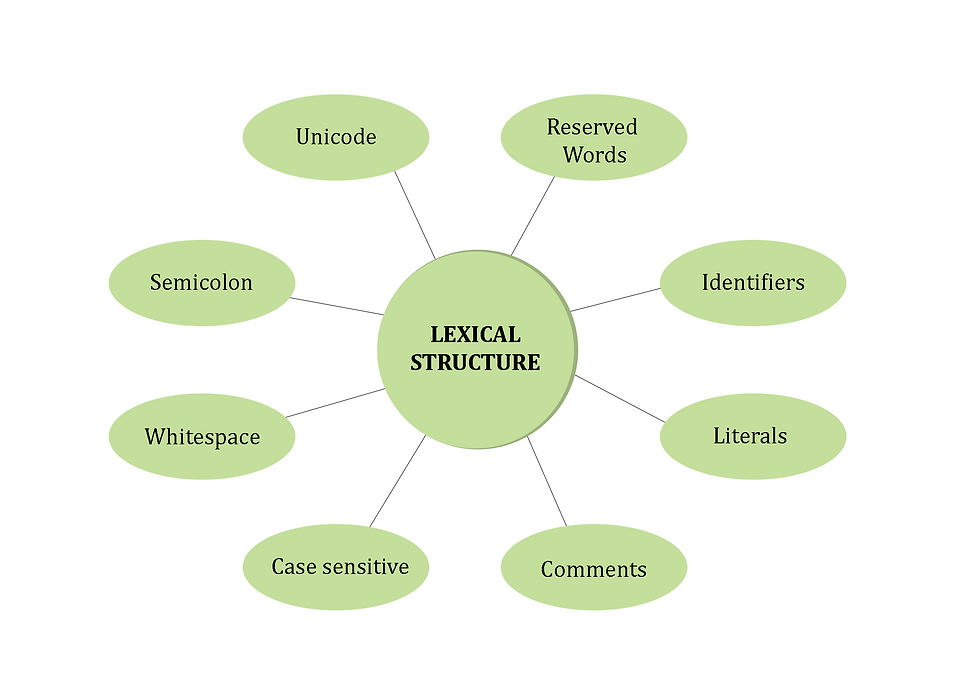
i) Unicode – JavaScript is written in Unicode. Unicode is an Information Technology standard for consistent representation, encoding and handling of text expressed in most of the world’s writing system. This means, we can use Emojis as variable names. We can also write identifiers in any language, say Japanese, Chinese, etc, with rules specified for defining identifiers.
ii) Semicolon – JavaScript uses semicolon at the end of each line to separate one programming statement from another. But, usage of semicolon is not mandatory. JavaScript does not throw any error if semicolons are omitted from the lines.
iii) White space – JavaScript does not consider white spaces and line breaks meaningful. Spaces and line breaks can be added in any fashion to increase the readability of the code or however the developer likes.
iv) Case sensitive – JavaScript is a case-sensitive language. This means that language keywords, variables, function names and identifiers should be typed with a consistent capitalization of letters. A variable named something is not equal to Something. Similarly, a keyword while should always be written as while and not WHILE, While or while, etc.
v) Comments – Comments are used to add hints, suggestions, or warnings to JavaScript code. This increases the code readability and understandability. JavaScript interpreter ignores the comment and do not execute them.
JavaScript comments can be accessed in two ways -
(1) Using “//” – In this, text of the same line is converted into comment using “//”. It supports single line comment.
Example –
function comment() {
// this is a single line comment
console.log(‘hello world’);
}
(2) Using “ /* */ “– This is the most flexible way of commenting code. Using this method, multiple lines of code can also be commented. We can also use it in the middle of a code to comment few words.
Example –
function comment() {
/* This is a
multiple line comment */
console.log (“Hello world”); /* Commenting few words */
}
vi) Literals – Literals are defined as values which are written in source code. For example, a number, a string, a Boolean, or more advanced constructs like object literals or array literals.
Example –
10
‘John’
true
[ ‘Apple’, ‘Banana’ ]
{name: ’Joey’, age: 30}
vii) Identifiers – An identifier is a sequence of characters that can be used to identify a variable, function or an object. There are certain rules to define an identifier. Some of the basic ones are –
(1) It can only start with letters, a dollar sign ($)or an underscore (_)
(2) It can contain digits. But it cannot start with a digit.
Some valid identifiers are – awesome, Awesome, $awesome9, _awesom2e
viii) Reserved words – JavaScript has some reserved words which cannot be used as identifiers or literals. List of reserved words of JavaScript are –
Break | case | catch | class | const | continue |
debugger | default | delete | do | else | export |
extends | false | finally | for | function | if |
imprt | in | instanceof | new | null | return |
static | super | switch | this | throw | true |
try | typeof | var | void | while | with |
yield | | | | | |
EXPRESSIONS
Expressions are very important in JavaScript. An expression can be defined as a valid unit of code that resolves to a value. According to the MDN documentation, there are various types of expressions in JavaScript. They are –

i) Arithmetic Expressions – These expressions evaluate to a numeric value. It uses arithmetic operators such as +, -, *, / and %. For example –
10 + 20; /* produces 30 as output */
20/4; /*produces 5 as output */
ii) String Expressions – These expressions evaluate to a string value. For example –
‘This is a test string.’ ;
“hello” + “World” + “!!” ;
iii) Logical Expressions – These are the expressions that evaluate to the Boolean value of true or false. They involve the usage of logical operators such as && (AND), || (OR) and ! (NOT). For example –
10 > 9 /* evaluates to boolean value true*/
10 < 9 /* Evaluates to boolean value false */
a===b && b===30 /* evaluates to true or false based on the values of a
and b */
iv) Primary Expressions – These are the expressions which are stand alone, such as literal values, keywords, variable values, etc. For example –
‘hello world’
true;
this;
v) Left-hand-side Expressions – These are the expressions that can appear on the left hand side of an assignment expression. For example –
// variables like i and total
i = 10;
total = 7;
vi) Assignment Expressions – These are the expressions which uses assignment operator (=) to assign a value to a variable. For example –
average = 11.5;
const var a = 100 + 2;
vii) Expressions with side effects – These are the expressions that result in a change or a side effect such as modification of a value of a variable through assignment operator, function call, incrementation or decrementation of a value of a variable. For example –
const sum = 20; //here sum is assigned the value of 20
sum++; // value of sum is incremented by 1
function modify(){
a+=10;
)
var a = 100;
modify(); // Modifies the value of a from 100 to 110
TYPES
JavaScript is a dynamic language. Variables in JavaScript are not directly associated with any particular data type. Any variable can be assigned and re-assigned value of any data type.
For example, the following code is possible in JavaScript, but not in many other popular languages such as C++, C, Java, etc.
let foo = 42; // now foo is a number
foo = ‘bar’; // Now foo is a string
foo = true; // Now foo becomes a Boolean
The set of types in JavaScript are mainly of two types: - Primitive and Objects.
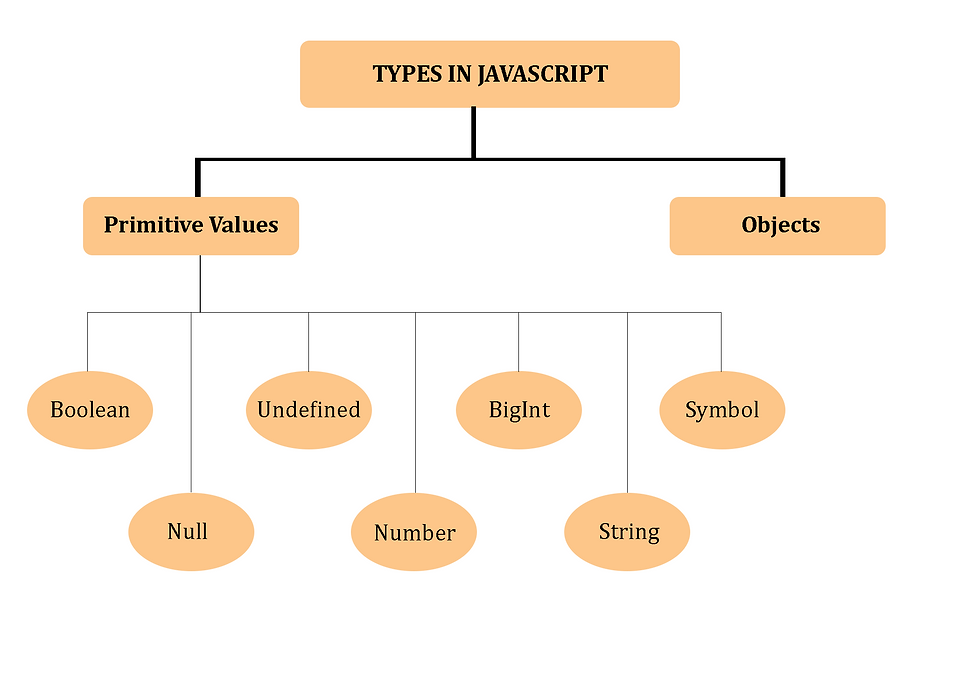
i) Primitive Values
These are the values which are immutable, i.e., values which cannot be changed. Following are the different types of primitive values –
Boolean Type – These are the logical types which can only have two values, either true or false. They are often used in conditional testing.
Example –
let x = 5;
let y = 10;
x==y; //return false
y>x; // returns true
Null Type – These are the types who represents no value at all. It holds exactly one value : NULL
Undefined Type – These are the types where variables have not been assigned any value.
Example –
let x;
console.log(x) //value of x is not defined
Numeric Type – ECMAScript in JavaScript has two built-in numeric types – Number and BigInt. Numbers in JavaScript can be written with or without decimals. JavaScript accepts both
Example –
let x1 = 10.22 //written with decinal
let x2 = 211 // written without decimal
BigInt Type – These are the types which can represent numbers with great precision. BigInts are safe to store and operate on large numbers which are beyond the limit of numberic types.
String Type – These are the types which are used to represent a textual data, written within double quotes. For example – “Hello” is a string type.
Symbol Type – Symbol is a unique data type which can be used as a key of an object’s property.
ii) Objects
In JavaScript, Objects are the collection of properties. They are written with curly braces {}. Objects in JavaScript are written as key: value pair, separated by commas..
Example –
let subject = { name : “Node.js”, language: “JavaScript”, application : “RESTApi” }
In the above example, subject is an object with three properties: name, language and application.
In this article we covered the following topics:-
These were the basic theory need to learn Node.js and JavaScript with full understanding. Next parts with cover the coding aspects of the same. You can refer to them via following links -
Thankyou.
Recent Posts
See AllNodemon is a very powerful and useful tool. Every time we make changes in our node.js program, we need to save it and then run it again...
Comments